Last modified: February 01, 2023
Lab A4.4 Draw Image
In this lab, you will create your own images by drawing directly in an image using the GreenfootImage class.
You can download a demo of this lab here.
Draw The Player Sprite
- Create a subclass of Actor named Player that will represent the player. Do not choose an image. You will be drawing the image in the constructor programatically.
- Create an default constructor for Player that does the following:
- Examine the GreenfootImage api and find the constructor that takes a width and height. Create a blank image with your choice of width and height.
- Draw an image for the player using the methods inside the GreenfootImage class such as fillRect, fillOval,...etc. Be creative!
- Note that the positions you pass to the drawing methods in GreenfootImage are relative to the upper left corner of the image NOT the world. For Example, drawing a rectangle at (0, 0) would place the rectangle in the upper left corner of your image. If you draw something at a position outside the bounds of the image, it will not be visible.
- You should draw at least 4 shapes in your image. In reality, to make a good picture you will need more than that.
- The image below is a simple example that meets the minimum requirements. I encourage you to try to make something significantly better than this.
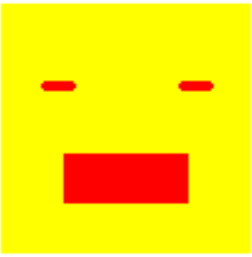
Add The Player
In the world class, add the player to the middle of the world.
Keyboard Movement
- Override the act() method so Player objects will move in the direction of the arrow while an arrow key is pressed.
- Player objects should stop moving if the key is released - only move while an arrow key is down.
- Player objects should never go even partially past any edge of the canvas.
Hunger Bar
- Create an instance variable in your Player class to hold the hunger level where 0 is fully satisfied and 100 is ravenous (max hunger)
- At the top edge of the player image you will add a hunger bar. The bar will originally be all green representing 0 hunger.
- Every 10 frames, hunger should increase by 1 and the hunger bar should reflect that by reduction.
- When hunger reaches 100, the player should remove itself from the world.
- The bar should be made up of green (on the left) and red (on the right). The red section should have a length proportional to the amount of hunger the player has out of 100.
Example of hunger bar showing 10 hunger:
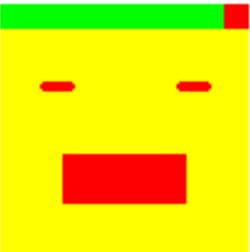
To update the Player's image, you have a few choices:
- Redraw the entire Player image and set Player's image to the newly drawn image during every animation frame (act method).
- In the Player's default constructor, you already drew the base image without the hunger bar. You can save this GreenfootImage into an instance variable. Then, instead of redrawing the entire player every time, you can make a copy of the base image and draw the hunger bar on top of it during every animation frame. In order make a copy of an image, use the constructor that accepts a GreenfootImage as the parameter. For example, if bob is a GreenfootImage that already exists, then you could make a copy of bob by saying:
GreenfootImage copy = new GreenfootImage(bob);
You would then draw the healthbar on the copy and set the Player's image to that image. This strategy can be very useful if you want to update text.
- Another method that would work in this case would be to take advantage of the fact that you are drawing completely over the same area each time, so you could simply draw both the green and red rectangles directly in the Player's current image, overwriting the previous bar. This works for the hunger bar, but would not work for text because you would still be able to see the old text underneath.
Spawn Food
- Create a subclass of Actor that represents food. In the constructor, assign it an image of food randomly. You can decide which images are possible.
- Each food object should have a food value that represents how much hunger is satisfied when it is eaten. Initialize the food value to an integer between 1 and 5.
- Food should have a small chance (like 1% or less) of spawning in a random location fully in the world each frame.
Eat
When the player touches food, the food should be removed and the player's hunger should be reduced by the food value of the food, down to a minimum of 0.
Game Over
- When the player is removed due to reaching 100 hunger, a message saying Game Over should appear in red text.
- To do this, you will need to make a class that has an image with text saying Game Over. Here are some options for doing that:
- Create a GreenfootImage using one of the constructors that takes a String and some other options and creates an image that says what the String says
- Create a blank GreenfootImage and use the drawString method. For this, you will also need to use the setColor and setFont methods and will need to investigate the Font class to see how to create a Font object.
- Once the game is over, no more food should spawn.
Submission
Zip your entire project folder and submit it below.
- Name your project folder
PX_LastName_FirstName_DrawImage
.
- Right click on your project folder and Send To -> Compressed (zipped) folder. On a mac compressing is similar, using a right click, but has a slightly different option.
- You should end up with a zip file named
PX_LastName_FirstName_DrawImage.zip
. For example if you were in 3rd period and named Michael Wang, then you would name the file P3_Wang_Michael_DrawImage.zip
- Upload the zip file.
You must Sign In to submit to this assignment